Integrating iOS SDK
Integrating the TestFairy SDK into your app helps you better understand how your app performs on real devices. It tells you when and how people are using your app, and provides you with any metrics you may need to optimize your user experience and code. You get to:
- Track app use.
- Handle crashes and report to server.
- Record screen video and other metrics.
- Understand user flow, using checkpoints.
- Grab NSLogs from client and report to server.
- Automatically update if a new build is available.
Adding the SDK
- Swift Package Manager
- Cocoapods
- Carthage
- Manual
Requires Xcode 12+. Screenshots taken from Xcode 13.1
- Select your project from the Xcode navigator to open your project's configuration.
- Make sure your project is selected from Project and Target list.
- Click the Package Dependencies Toolbar item.
- Click the '+' icon to add a package.
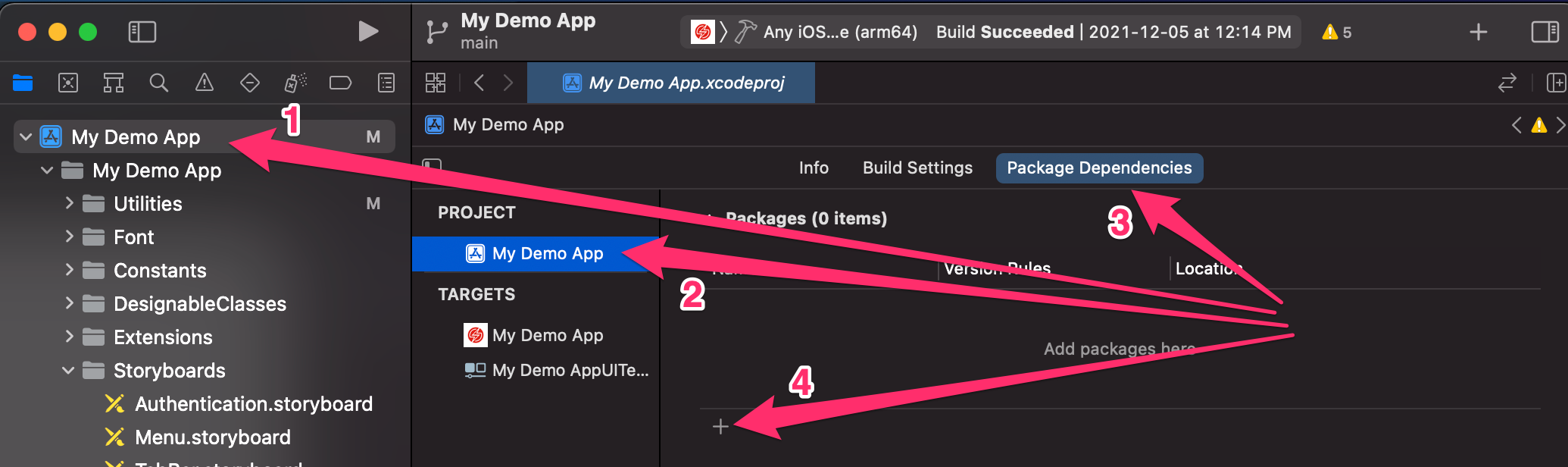
- In the newly opened dialog search for the TestFairy package repository using the URL: https://github.com/testfairy/testfairy-ios-sdk-swift-package in the top right search bar.
- Click the Add Pacakge button.
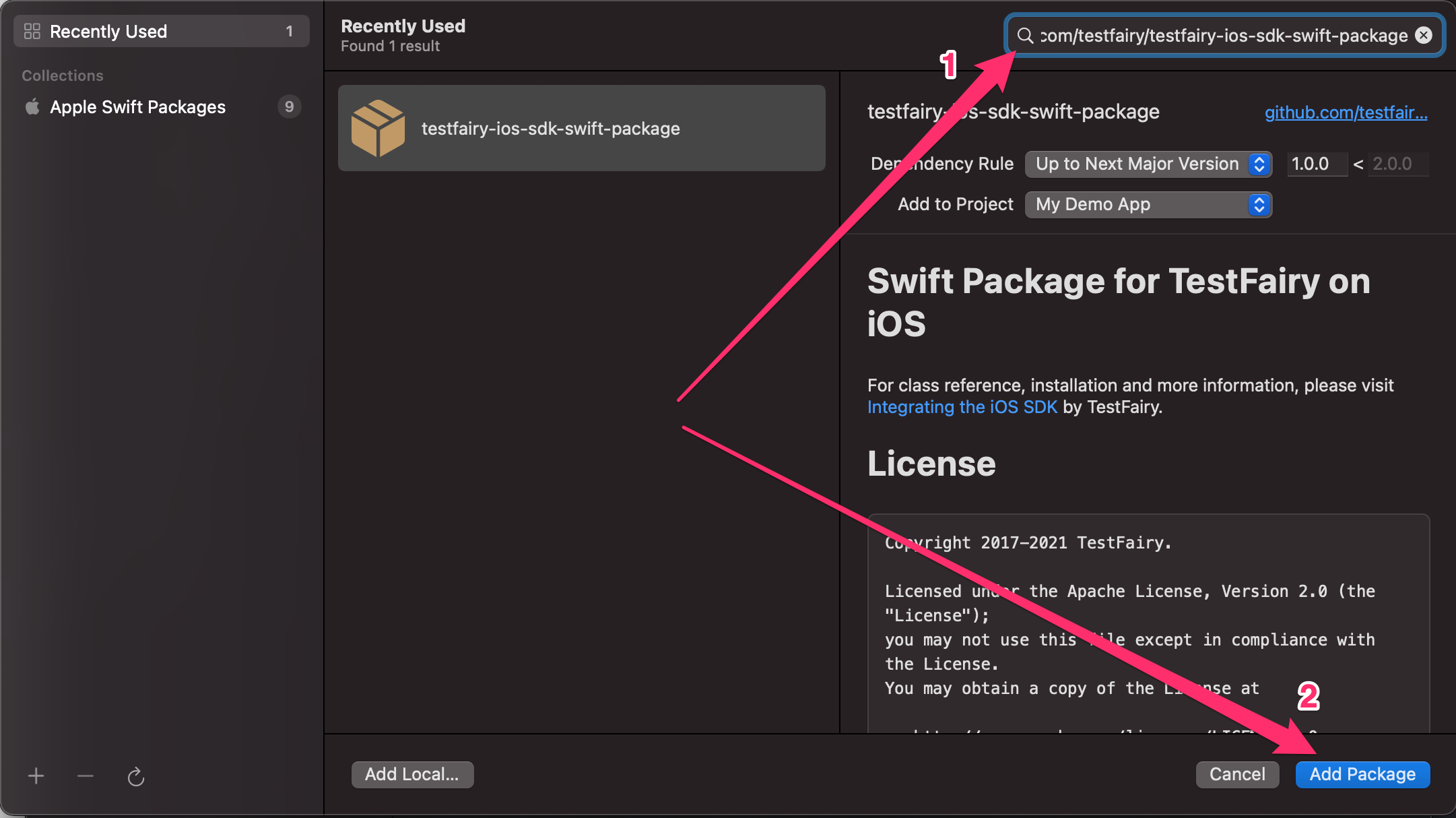
- After the package has been downloaded, in the newly opened dialog, make sure the TestFairy package is selcted in the "Package Product" column
- Make sure the right target is selected in the "Add to target" column
- Click the Add Pacakge button
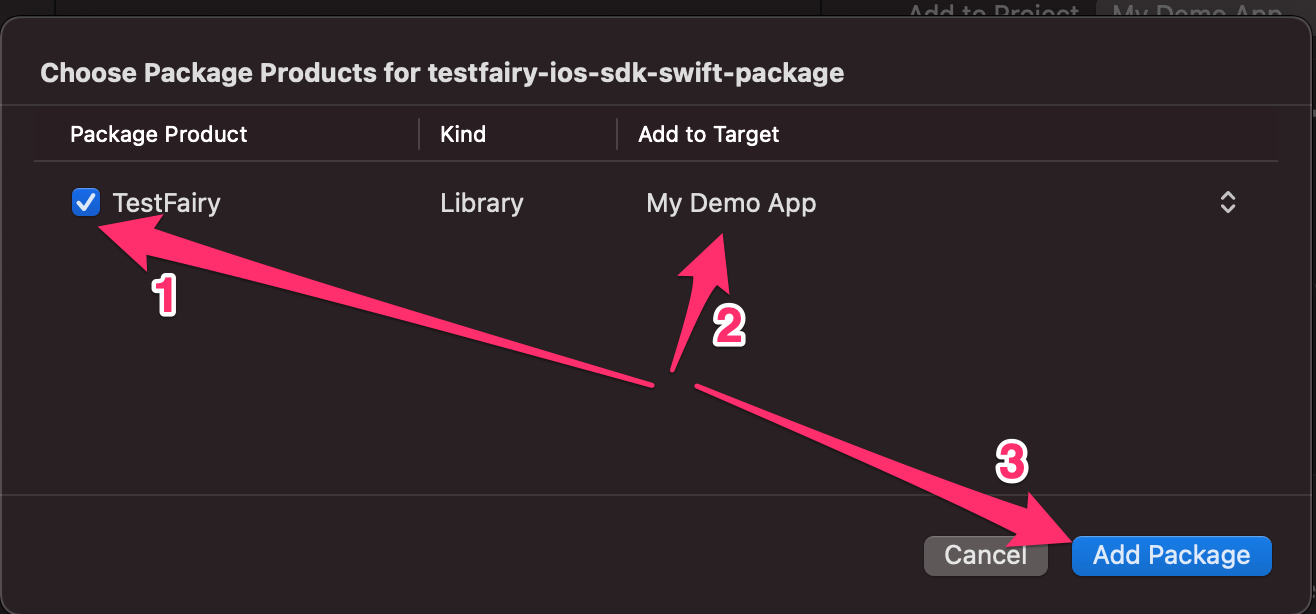
- Add the TestFairy pod to your Podfile by inserting the following line where applicable:
pod 'TestFairy'
- Run the
$ pod install
command to install theTestFairy
dependency.
- Add
binary "https://app.testfairy.com/sdk/ios/carthage.json"
to your Cartfile. - Run
carthage update
. - On your application targets’ “General” settings tab, in the “Linked Frameworks and Libraries” section, drag and drop the TestFairy framework from the [Carthage/Build][] folder on disk.
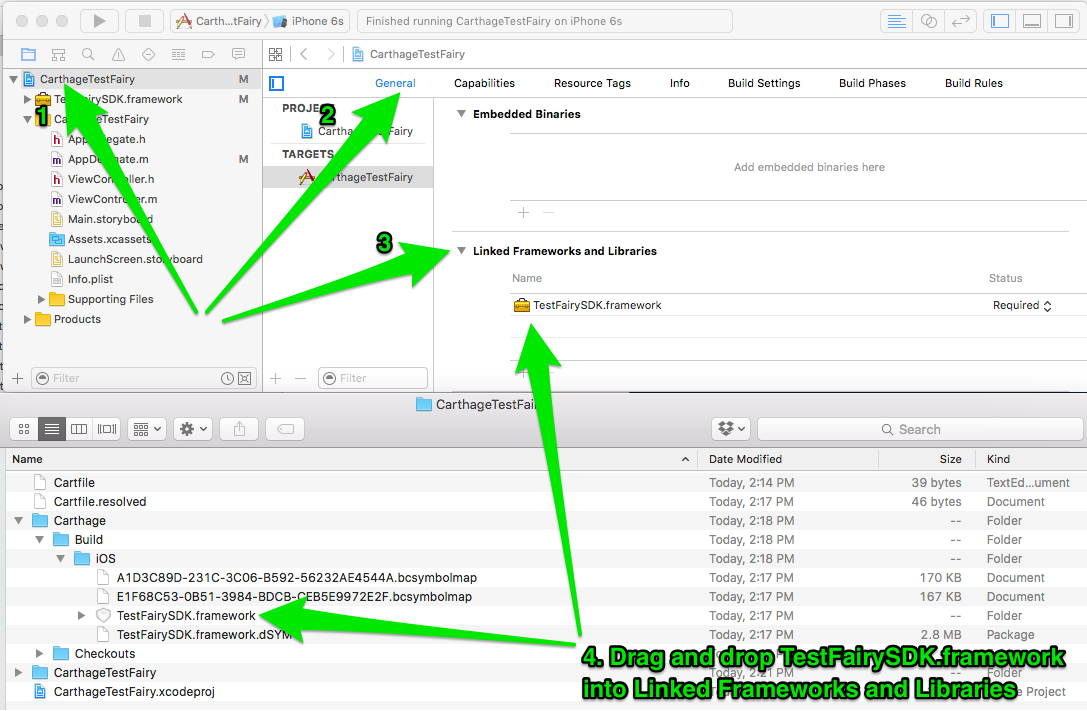
- On your application targets’ “Build Phases” settings tab, click the “+” icon and choose “New Run Script Phase”. Create a Run Script in which you specify your shell (ex: bin/sh), add the following contents to the script area below the shell:
/usr/local/bin/carthage copy-frameworks
${SRCROOT}/Carthage/Build/iOS/TestFairySDK.framework
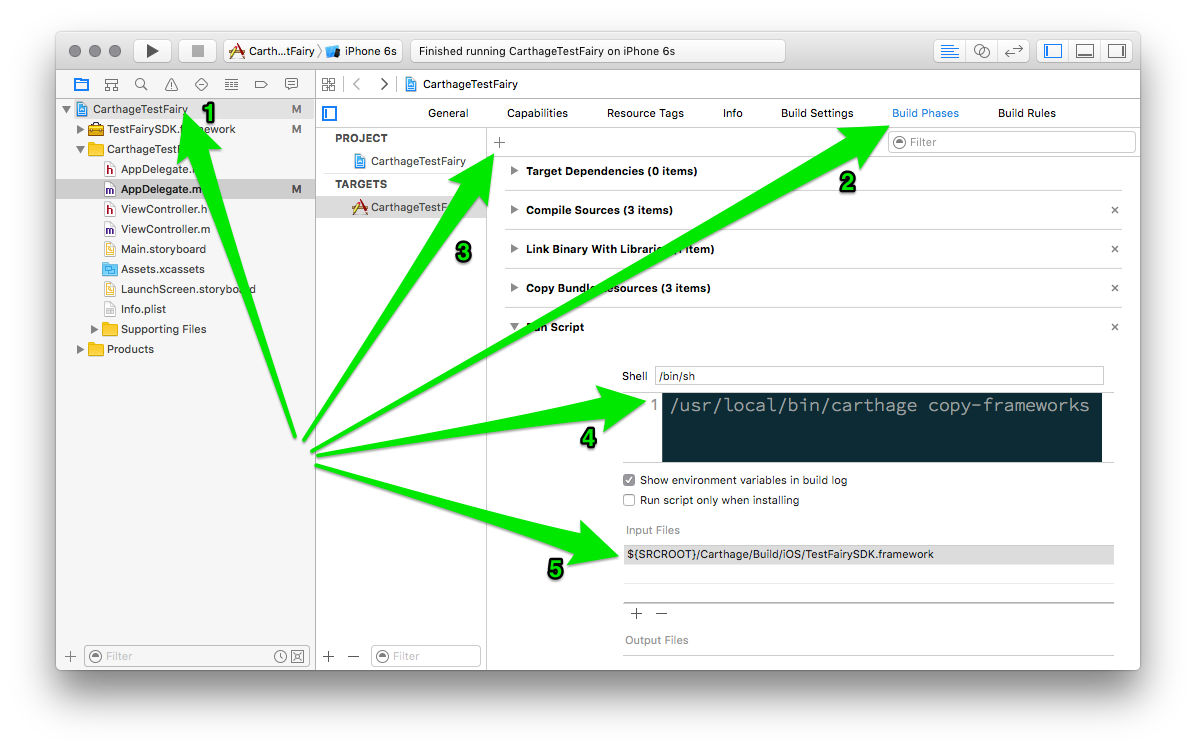
- Download the framework from our Download page.
- Unzip files and drag them into your project tree.
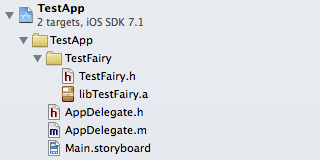

- Add the following framework:
- In Xcode, select the project file from the project navigator, on the left side of the project window.
- Show Projects and Target List.
- In the project settings editor, select the target to which you would like to add frameworks.
- Select the “Build Phases” tab, and click the small triangle next to “Link Binary With Libraries” to view all of the frameworks in your application.
SystemConfiguration.framework
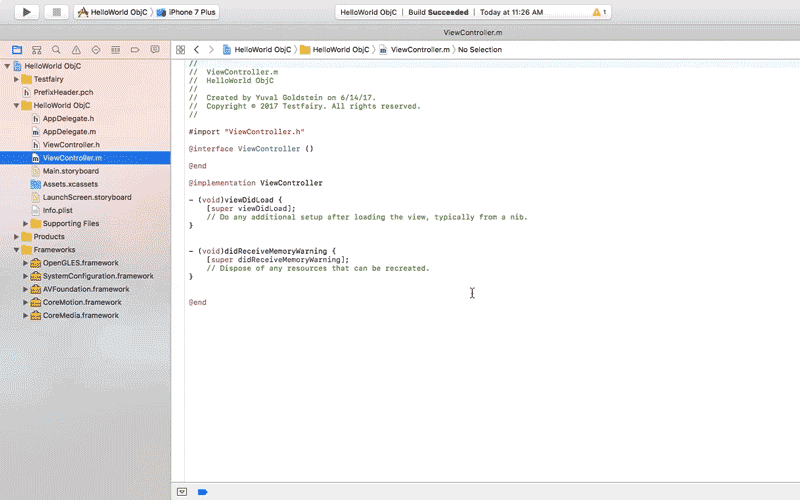
Initializing the SDK
- Objective C
- Swift
-
Open your AppDelegate.m file.
-
Add this code to your app:
#import "TestFairy.h"
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[TestFairy begin:@"SDK-u6qN9qXN"];
// below of the rest of the didFinishLaunchingWithOptions method
// ...
}
- Create an Objective-C bridging header
Since this process only needs to be done once per project, if you have already done so, just update your bridging header file.
- Right-click on your project, select New File...
- Select Header File.h
- Save as Bridging.h in your project
- Click on Bridging.h to open it in editor
- Add the following line to the code:
#import "TestFairy.h"
If framework wasn't uploaded manually please try:
#import "TestFairy/TestFairy.h"
- Click on your project
- Select Build Settings tab
- Select the "All" filter, in order to find Swift Compiler - General: Objective-C Bridging Header
- Edit Swift Compiler - Code Generation: Objective-C Bridging Header (double-click to edit).
- Drag "Bridging.h" from the source tree onto the edit box opened
-
Open your AppDelegate.swift file.
-
Add this code to your app:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
TestFairy.begin("SDK-u6qN9qXN")
// below of the rest of the didFinishLaunchingWithOptions method
// ...
return true
}
Using PencilKit for Better Feedback
You can give your users a better set of tools to markup any screenshots provided during feedback by adding PencilKit to your project. Simply add the PencilKit.framework to your project.
This requires iOS 13 and Xcode 11.
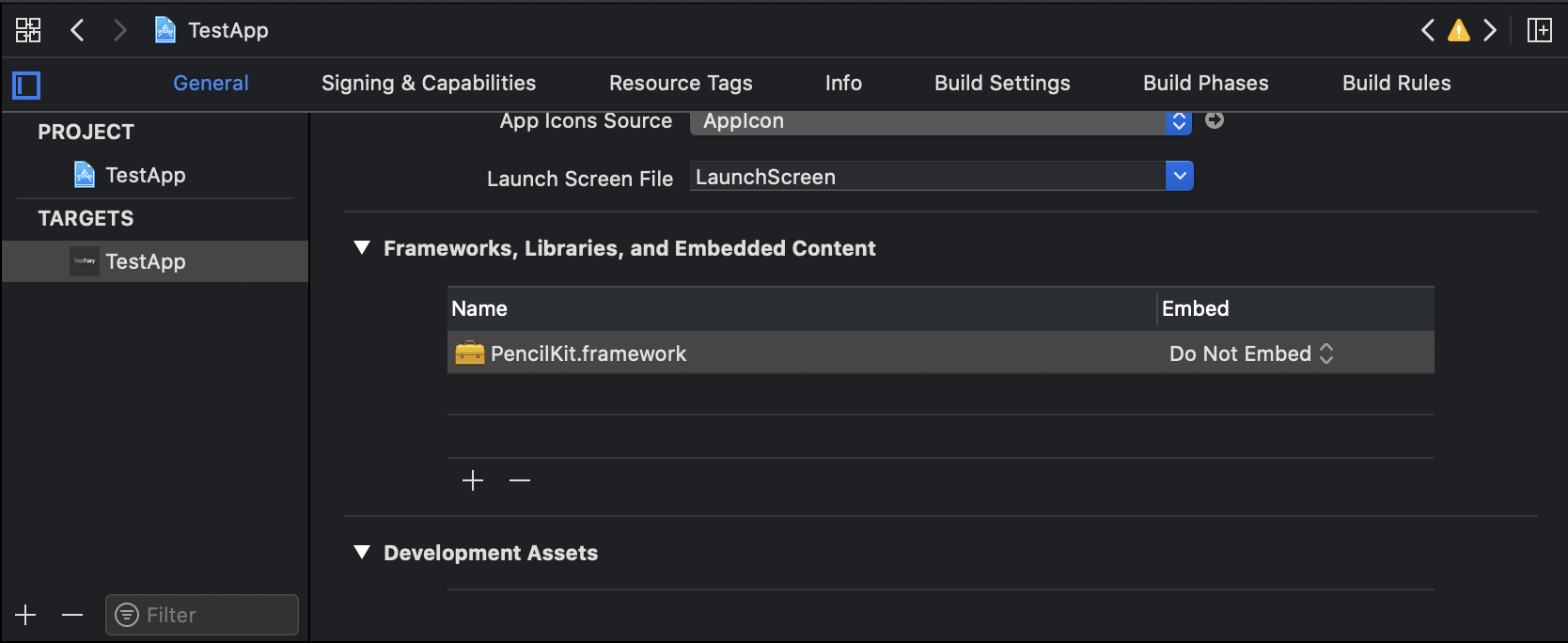
If a screenshot is attached to the feedback, your users can edit the screenshot by tapping on it and using PencilKit to mark it up.
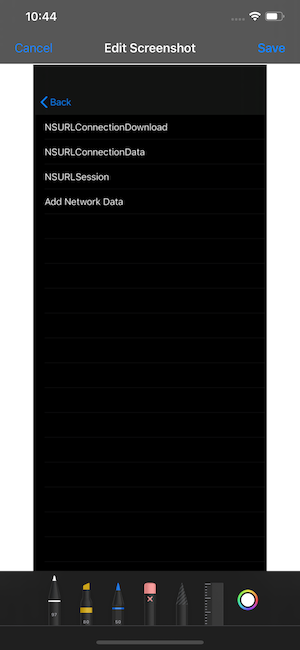