Hiding Sensitive Data
TestFairy offers a valuable feature that allows developers to conceal sensitive information from recorded sessions, ensuring that sensitive data, such as credit card information, remains protected during testing and debugging.
For example, you might want to prevent all information related to credit card data from appearing in the session:
- Android
- iOS
- React Native
- Nativescript
- Xamarin
To hide a view from video, all you need to do is the following:orReplace
TestFairy.hideView(Integer.valueOf(R.id.my_view));
TestFairy.hideView(View myView);
R.id.my_view
with the identifier of the view you wish to hide. Review the full example below:Examplepublic class MyActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.my_activity);
TestFairy.hideView(findViewById(R.id.credit_card));
}
}
To hide a view from video, all you need to do is call the static instance method hideView in the TestFairy class:Example
UIView *view = ...
[TestFairy hideView:view];
@interface MyViewController : UIViewController {
IBOutlet UITextField *usernameView;
IBOutlet UITextField *creditCardView;
IBOutlet UITextField *cvvView;
}
@implementation MyViewController
- (void)viewDidLoad {
[super viewDidLoad];
[TestFairy hideView:creditCardView];
[TestFairy hideView:cvvView];
}
To hide views from your recorded session, you must pass a reference to a view to TestFairy. First, give the element to be hidden as a ref attribute. For example:Next, in a component callback, such as componentDidMount, pass the reference ID back to TestFairy by invoking hideView.Example
<Text ref="instructions">This will be hidden</Text>
const TestFairy = require('react-native-testfairy');
var MyComponent = React.createClass({
componentDidMount: function() {
TestFairy.hideView(this.refs.instructions);
},
render: function() {
return (<Text ref="instructions">This will be hidden</Text>);
}
});
TestFairySDK.hideView(view);
// in Nativescript
import { TestFairySDK } from 'nativescript-testfairy';
// in Javascript
var TestFairySDK = require('nativescript-testfairy').TestFairySDK;
TestFairySDK.hideView(view);
TestFairy.HideView (View view) - on Android
TestFairy.HideView (UIView view) - on iOS
// Be sure to import TestFairy
using TestFairyLib;
// On Android
View view = ...
TestFairy.HideView (view);
// On iOS
UIView view = ...
TestFairy.HideView (view);
Example
Below is a screen taken from a demo video: on the left, you can see what an app usually looks like; on the right is a screenshot taken with the Card Number EditText hidden by testfairy-secure-viewid
.
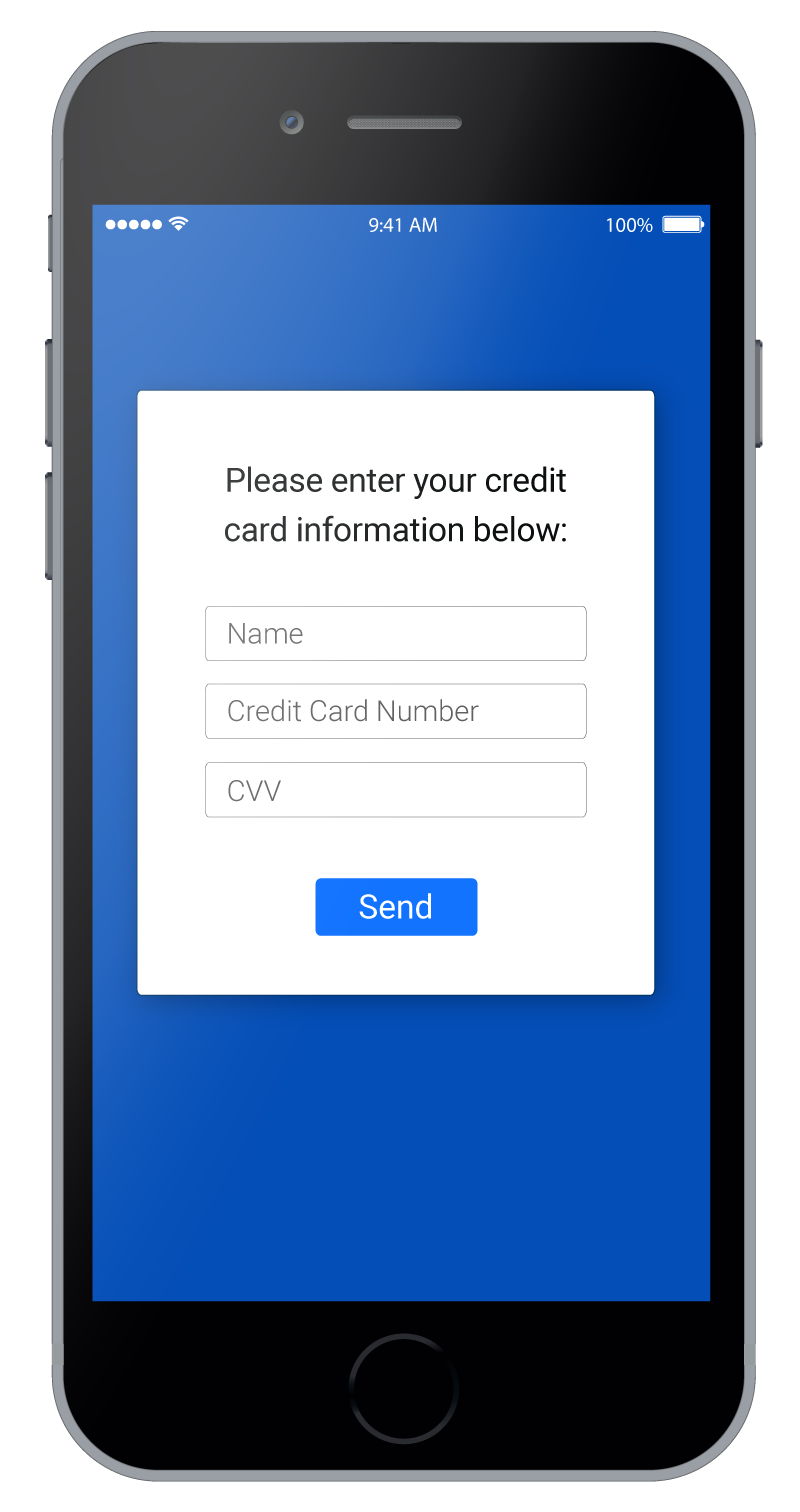
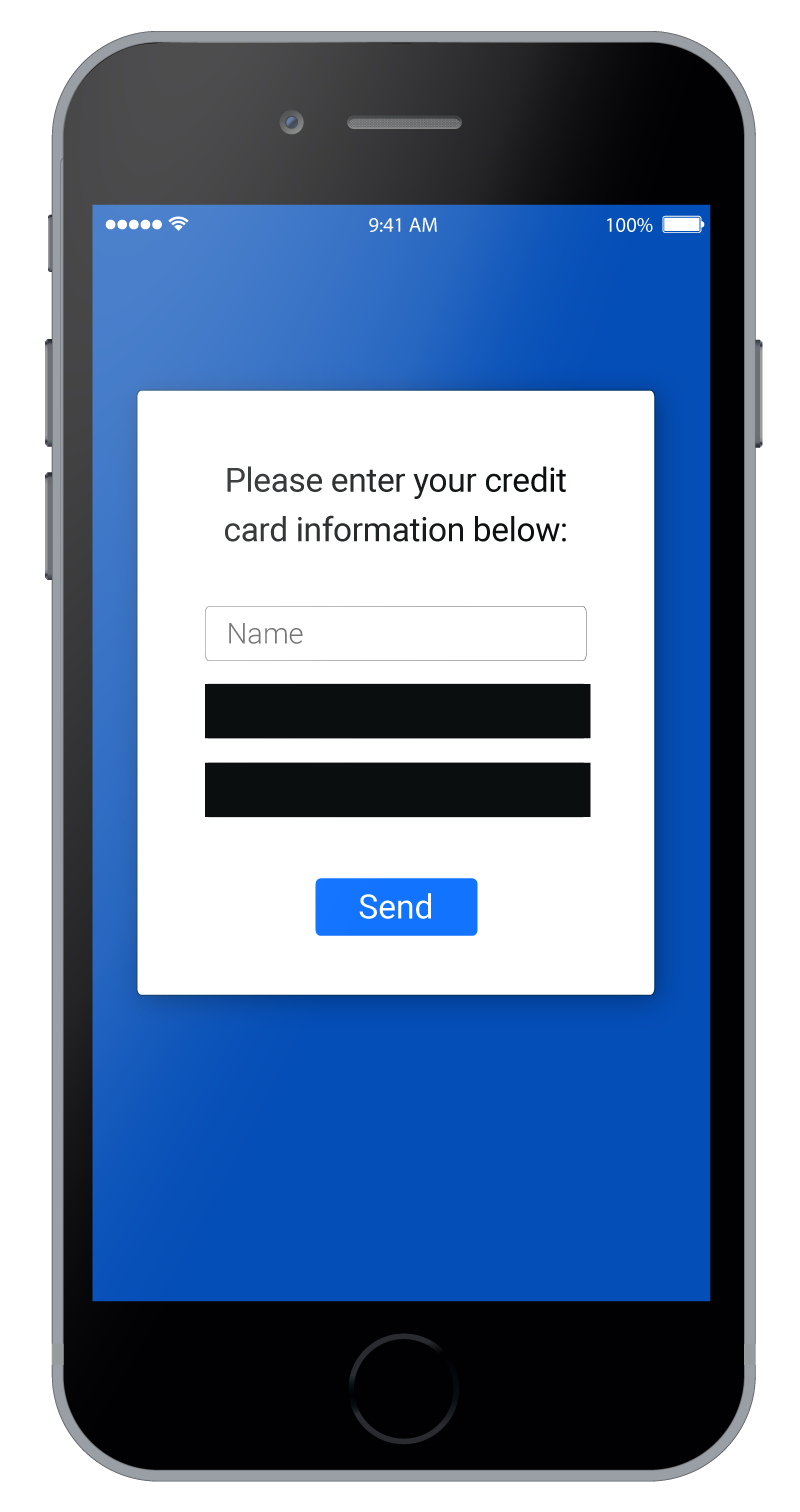
note
- Hidden views are automatically removed from the video before being sent to TestFairy's servers, ensuring that sensitive data is never captured or exposed.
- Developers can hide multiple views within a session to protect various sensitive elements in the application.
- It is permissible to add the same view multiple times for hiding without any additional checks.